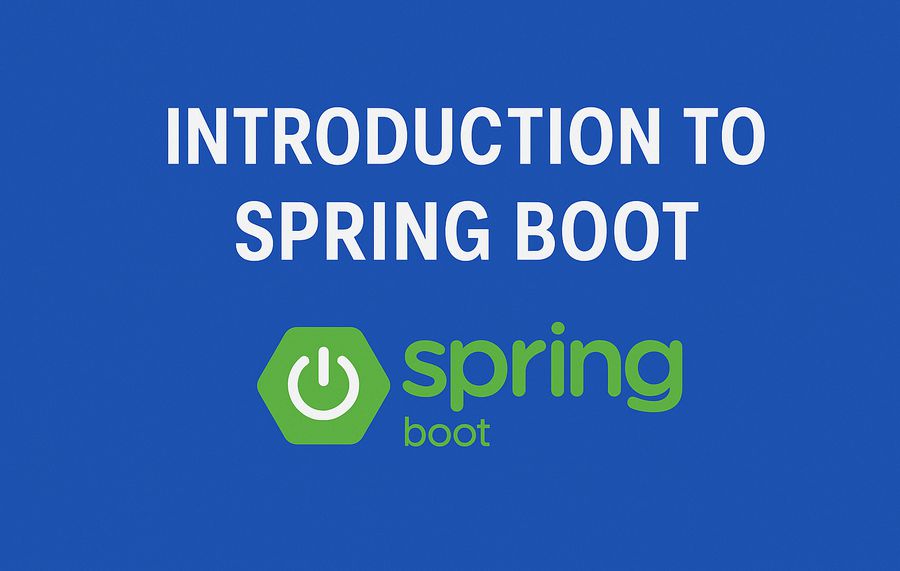
Introduction to Spring Boot: Simplifying Java Application Development
Introduction to Spring Boot
Spring Boot is a powerful framework that simplifies the development of stand-alone, production-ready applications using the Spring ecosystem. It provides a convention-over-configuration approach, reducing the need for extensive setup and boilerplate code. With Spring Boot, developers can focus on building features rather than managing infrastructure.
Why Choose Spring Boot?
Spring Boot is widely adopted due to its simplicity, flexibility, and robust features. Here are some key reasons why developers prefer it:
-
Minimal Configuration: Spring Boot eliminates complex XML configurations, allowing developers to start projects quickly.
-
Embedded Server Support: Applications can run independently without requiring an external server like Tomcat or Jetty.
-
Auto-Configuration: It automatically configures components based on project dependencies.
-
Microservices-Friendly: Ideal for building distributed applications and microservices.
-
Spring Boot Starters: Pre-configured dependencies simplify project setup.
-
Production-Ready Features: Integrated support for monitoring, health checks, and security.
Core Features of Spring Boot
-
Auto Configuration - Reduces the need for manual setup.
-
Spring Boot Starters - Predefined dependencies for common use cases.
-
Embedded Servers - Includes Tomcat, Jetty, and Undertow for seamless deployment.
-
Spring Boot Actuator - Provides built-in monitoring and management tools.
-
Spring Boot CLI - Allows running and testing applications from the command line.
-
Spring Boot Security - Simplifies authentication and authorization.
Getting Started with Spring Boot
To create a Spring Boot application, follow these simple steps:
-
Set Up the Project: Use Spring Initializr to generate a project with the necessary dependencies.
-
Include Dependencies: Add Spring Boot starters (e.g.,
spring-boot-starter-web
for REST APIs). -
Create the Main Application Class:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
-
Run the Application: Use
mvn spring-boot:run
or execute themain
method in your IDE.
Spring Boot Starters
Spring Boot provides various starters to simplify dependency management. Some common ones include:
-
spring-boot-starter-web
- For building RESTful web services. -
spring-boot-starter-data-jpa
- For database integration with JPA/Hibernate. -
spring-boot-starter-security
- For securing applications. -
spring-boot-starter-test
- For unit and integration testing. -
spring-boot-starter-thymeleaf
- For template-based web applications.
Conclusion
Spring Boot is a game-changer in Java development, making it easier to create robust, scalable applications. With its auto-configuration, embedded server support, and production-ready features, it streamlines development and enhances productivity. Whether building a simple web app or a complex microservices architecture, Spring Boot provides the tools necessary to succeed.