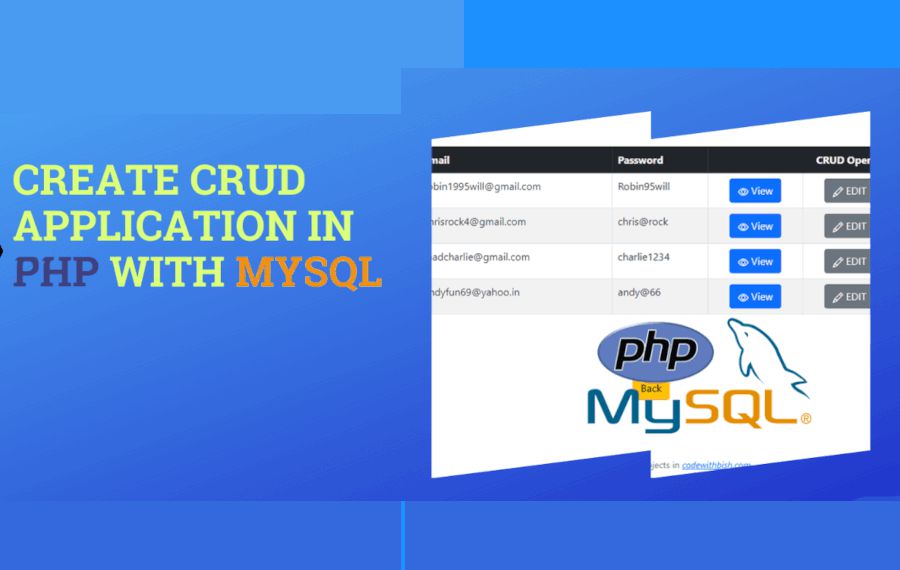
REST API CRUD using PHP and MySQL
In this tutorial, we will learn how to create a simple RESTful API for CRUD (Create, Read, Update, Delete) operations and the necessary HTTP Verbs. We will use functional programming approach in PHP
2. Database & Table Configurations
3. dbconfig.php
4. create.php
5. index.php
5. update.php
6. delete.php
1. Project Structure
This is going to be the file structure of our project. Placed in location of the folder under "/var/www/html"
2. Database & Table Configuration
First we need to install MySQL database, if not Install Here.
Then we create a database, we can use phpMyAdmin or using the terminal. In this tutorial we are going to use terminal.
- Login to MySQL
mysql -u root -p
- Create new database called "php_crud_api"
CREATE DATABASE php_crud_api;
- Make use of the database
USE php_crud_api;
- Create table.
Run the following script to create products table.
`product_id` int(11) NOT NULL,
`product_name` varchar(30) NOT NULL,
`product_price` int(11) NOT NULL
);
It will create products table with default of engine InnoDB
and you can verify using the following script
SHOW tables;
3. dbconfig.php
We will create a configuration file "dbconfig.php" where we will store our database configurations we will use.
<?php $databaseHost = 'localhost'; $databaseUsername = 'root'; $databasePassword = 'xxxxxxxxxx'; $databaseName = 'php_crud_api'; $conn = mysqli_connect($databaseHost, $databaseUsername, $databasePassword, $databaseName); if (!$conn){ die("Database Connection Failed" . mysqli_error($conn)); }
4. create.php
create a file "create.php" that will accept form inputs of "id, name, price" and store them in products table.
<?php header('Content-Type: application/json')
header('Access-Control-Allow-Methods: POST');
include("dbconfig.php"); $data = json_decode(file_get_contents('php://input'), true); $id = $data['id']; $name = $data['name']; $price = $data['price']; $CreateSql = "INSERT INTO products (product_id, product_name, product_price) VALUES ('$id', '$name', '$price')"; $res = mysqli_query($conn, $CreateSql) or die(mysqli_error($conn)); if($res){ $response = array("message"=>"Product inserted successfully", "status"=>true); echo json_encode($response, JSON_PRETTY_PRINT); }else{ $response = array("message"=>"Failed to insert Product", "status"=>false); echo json_encode($response, JSON_PRETTY_PRINT); } ?>
we use Postman to create the products
we set HTTP VERB as "POST", and input we select raw and category "JSON". After inserting successfully you will be displayed with a success response as shown below
5. index.php
create a file "index.php" that will read all records from the products table and display in JSON format.
<?php header('Content-Type: application/json'); include("dbconfig.php"); $result = mysqli_query($conn, "SELECT * FROM products") or die(mysqli_error($conn)); $row = mysqli_fetch_all($result, MYSQLI_ASSOC); echo json_encode($row, JSON_PRETTY_PRINT); ?>
we use Postman to read the products
we set HTTP VERB as "GET", it will diplay array of product objects
6. update.php
create a file "update.php" that will update one record based on product_id.
header('Content-Type: application/json');
header('Access-Control-Allow-Methods: PUT');
include("dbconfig.php");
$data = json_decode(file_get_contents('php://input'), true);
$id = $data['id'];
$name = $data['name'];
$price = $data['price'];
$updateSql = "UPDATE products SET product_name = '$name', product_price = '$price' WHERE product_id = '$id'";
$res = mysqli_query($conn, $updateSql) or die(mysqli_error($conn));
if($res){
$response = array("message"=>"Product Updated successfully", "status"=>true);
echo json_encode($response, JSON_PRETTY_PRINT);
}else{
$response = array("message"=>"Failed to Update Product", "status"=>false);
echo json_encode($response, JSON_PRETTY_PRINT);
}
?>
7. delete.php
create a file "delete.php" that will delete one record based on product_id.
header('Content-Type: application/json');
header('Access-Control-Allow-Methods: DELETE');
include("dbconfig.php");
$data = json_decode(file_get_contents('php://input'), true);
$id = $data['id'];
$query = "DELETE FROM products WHERE product_id = '$id'";
$res = mysqli_query($conn, $query) or die(mysqli_error($conn));
if($res){
$response = array("message"=>"Product Deleted successfully", "status"=>true);
echo json_encode($response, JSON_PRETTY_PRINT);
}else{
$response = array("message"=>"Failed to Delete Product", "status"=>false);
echo json_encode($response, JSON_PRETTY_PRINT);
}
?>