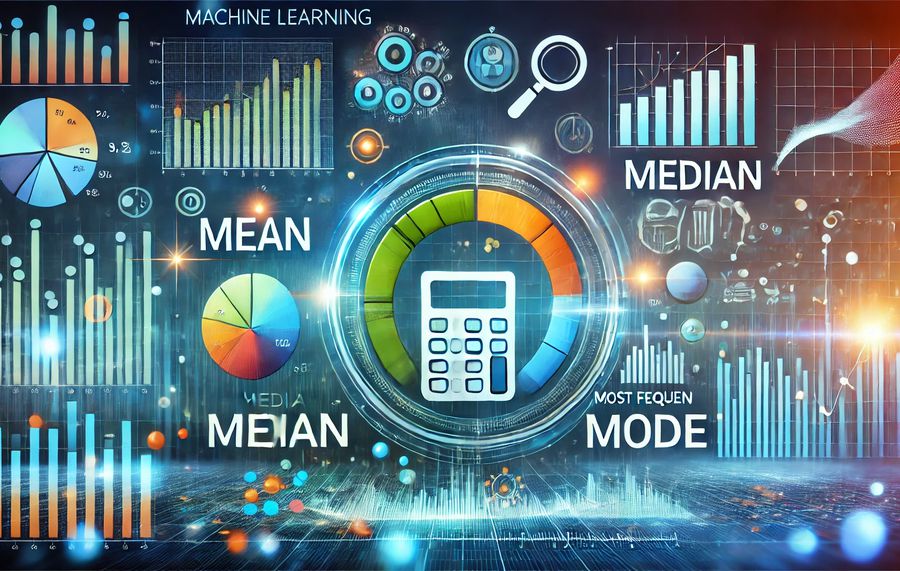
Machine Learning - Mean Median Mode
Understanding Mean, Median, and Mode in Machine Learning
In the realm of machine learning, data is king. Whether you're training a model or analyzing results, understanding statistical measures is crucial for making sense of your data. Three fundamental concepts that frequently come into play are Mean, Median, and Mode. Let's explore these measures and how they apply to machine learning with some illustrative examples.
Mean: The Average Value
The mean, often referred to as the average, is calculated by summing all the values in a dataset and then dividing by the number of values. It provides a central value for the dataset, giving a quick snapshot of the data's overall tendency.
Example 1: Predicting House Prices
Imagine you're building a machine learning model to predict house prices in a neighborhood. You collect the following data for house prices (in thousands of dollars): 200, 250, 300, 350, and 400.
To find the mean price:
- Sum the values: 200 + 250 + 300 + 350 + 400 = 1500
- Divide by the number of values: 1500 / 5 = 300
So, the mean house price is $300,000. This value helps in understanding the typical house price in the area, which can be crucial for setting benchmarks or evaluating model performance.
Example 2: Assessing Student Scores
Suppose you have a dataset of student scores from a test: 80, 85, 90, 95, and 100. To find the mean score:
- Sum the values: 80 + 85 + 90 + 95 + 100 = 450
- Divide by the number of values: 450 / 5 = 90
The mean score is 90. This information can be used to evaluate the overall performance of students and adjust your model’s predictions or recommendations.
Median: The Middle Value
The median is the middle value in a dataset when the values are sorted in ascending or descending order. If there is an even number of observations, the median is the average of the two middle values. The median is particularly useful for understanding the central tendency of a dataset, especially when the data is skewed or contains outliers.
Example 1: Evaluating Salary Data
Consider you’re analyzing salaries in a company: $50,000, $55,000, $60,000, $70,000, and $200,000. Sorting these values gives us the same sequence, and the middle value is $60,000.
The median salary is $60,000. Unlike the mean, which can be heavily influenced by the $200,000 outlier, the median provides a better sense of the typical salary within the company.
Example 2: Analyzing Response Times
If you're looking at the response times of a web application in milliseconds: 120, 130, 140, 150, and 200. Sorting the values, the median response time is 140 milliseconds. This measure helps you understand the typical user experience without the skew of a potential slow outlier.
Mode: The Most Frequent Value
The mode is the value that appears most frequently in a dataset. A dataset may have one mode, more than one mode, or no mode at all. The mode is particularly useful for categorical data where we want to identify the most common category.
Example 1: Popular Product Choices
Imagine you're analyzing customer preferences for a new product and collect the following data on the product choices: A, B, A, C, B, A. The mode here is A, as it appears more frequently than the other choices. This insight helps in identifying which product option is most popular among customers.
Example 2: Survey Responses
In a survey about preferred work-from-home days, the responses are: Monday, Wednesday, Friday, Monday, Tuesday, Monday. The mode is Monday. This indicates that Monday is the most preferred work-from-home day among respondents.
Conclusion
Mean, median, and mode are foundational concepts in statistics that play a crucial role in data analysis and machine learning. The mean provides an average, the median offers a middle point unaffected by outliers, and the mode reveals the most common value. Understanding these measures helps in interpreting data correctly and making informed decisions, whether you're developing predictive models or analyzing survey results.
By leveraging these statistical tools, you can enhance your machine learning projects, ensuring more accurate and meaningful insights from your data.
Example: Analyzing and Preparing Data for Machine Learning
Scenario
Suppose you are working with a dataset of student exam scores and want to perform some basic statistical analysis to prepare the data for a machine learning model. You'll use the Mean, Median, and Mode to understand the central tendency of the scores and to handle any anomalies in the data.
Dataset
Let's use a small dataset of student scores: [78, 82, 85, 90, 95, 100, 100, 100, 105, 110]
Steps
-
Install Necessary Packages
If you haven't installed NumPy and Pandas, you can do so using pi
pip install numpy pandas
2. Load and Analyze Data Using Python
Here's a Python script to calculate Mean, Median, and Mode, and to visualize the data using Pandas and NumPy.
import numpy as np
import pandas as pd
from scipy import stats
# Sample dataset
scores = [78, 82, 85, 90, 95, 100, 100, 100, 105, 110]
# Convert to a Pandas Series
scores_series = pd.Series(scores)
# Calculate Mean
mean_score = np.mean(scores_series)
print(f"Mean Score: {mean_score}")
# Calculate Median
median_score = np.median(scores_series)
print(f"Median Score: {median_score}")
# Calculate Mode
mode_score = stats.mode(scores_series)
print(f"Mode Score: {mode_score.mode}, Count: {mode_score.count}")
# Visualize the data distribution
import matplotlib.pyplot as plt
plt.hist(scores_series, bins=range(70, 120, 10), edgecolor='black')
plt.axvline(mean_score, color='r', linestyle='dashed', linewidth=1, label=f'Mean: {mean_score}')
plt.axvline(median_score, color='g', linestyle='dashed', linewidth=1, label=f'Median: {median_score}')
plt.axvline(mode_score.mode, color='b', linestyle='dashed', linewidth=1, label=f'Mode: {mode_score.mode}')
plt.xlabel('Scores')
plt.ylabel('Frequency')
plt.title('Distribution of Student Exam Scores')
plt.legend()
plt.show()
-
Explanation:
- Mean: Computed using
np.mean()
, which gives the average score. - Median: Computed using
np.median()
, which provides the middle value when the data is sorted. - Mode: Computed using
stats.mode()
, which finds the most frequent score in the dataset.
- Mean: Computed using
-
Interpreting Results
Running the above script will provide you with the Mean, Median, and Mode of the exam scores, along with a histogram that visually represents these statistics:
- Mean Score: Gives you an idea of the average performance.
- Median Score: Shows the middle point of the data, providing insight into the central tendency without being skewed by outliers.
- Mode Score: Indicates the score that occurs most frequently, which can help in understanding common performance levels.
The histogram will have vertical dashed lines representing the Mean, Median, and Mode, making it easy to see how these measures compare visually.